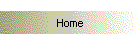
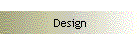
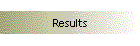
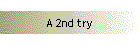
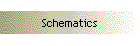
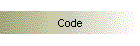
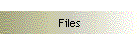
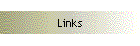
| | //main.c
//TEST UART AND SRAM
#include <mega128.h>
//#include <stdlib.h>
#include <stdio.h> //sprintf
#include <delay.h>
#include "serial.h"//my serial functions
#include "sta013.h"
#include "ui.h"//user interface
//set lcd_port 0x18 for port B
#asm
.equ __lcd_port=0x18
#endasm
#include <lcd.h> // LCD driver routines
#ifndef begin
#define begin {
#define end }
#endif
//UART using UART1 on PORTD[2..3]
void initialize(void);
void main(void)
begin
unsigned char done;
initialize();
S_Printf("\n\rWelcome to Serial MP3 Player\n\r");
S_Printf("Version 1.0 by Zhi-Hern Loh\n\r");
S_Printf("User interface is as follows\n\r");
S_Printf("Button|Function\n\r");
S_Printf(" 0 Start playback\n\r");
S_Printf(" 1 Stop playback\n\r");
S_Printf(" 2 Volume Up\n\r");
S_Printf(" 3 Volume Down\n\r");
STA013_Init();
/*
//STA013_Test_Serial_Interface();
S_Printf("STA initialized, now playing test song\n\r");
//STA013_Play_MP3();
S_Printf("Test song complete\n\r");
*/
//Test Buttons
done=0;
vol_attn_l = vol_attn_r = 0;
/*
while(!done)
begin
debounce();
if (New_Butnum)
begin
switch(Current_Butnum)
begin
case Btn_Start:
lcd_gotoxy(0,1);lcd_putsf("Start");
break;
case Btn_Stop:
lcd_gotoxy(0,1);lcd_putsf("Stop");
break;
case Btn_Vol_Up:
lcd_gotoxy(0,1);lcd_putsf("Vol up");
if (vol_attn_l>0) vol_attn_l--;
if (vol_attn_r>0) vol_attn_r--;
STA013_Write(DLA, vol_attn_l);
STA013_Write(DRA, vol_attn_r);
break;
case Btn_Vol_Dn:
lcd_gotoxy(0,1);lcd_putsf("Vol dn");
if (vol_attn_l<255) vol_attn_l++;
if (vol_attn_r<255) vol_attn_r++;
STA013_Write(DLA, vol_attn_l);
STA013_Write(DRA, vol_attn_r);
break;
case (1<<4):
done=1;
break;
default:
break;
end
Clr_Butnum;
end
delay_ms(30);
end
lcd_gotoxy(0,1);lcd_putsf(" "); */
while(1)
begin
/*
S_Printf("Testing 1 sec timer\n\r");
PORTE=PORTE&0x00;
SEC_TIMER_ISR_ON;
SEC_COUNTER_ON;
S_Printf("TIM0 activated\n\r");
*/
S_Printf("Ready for Xmodem transfer\n\r");
S_Printf("Press Btn 0 to start Xmodem\n\r");
//pause();
done=0;
while(!done)
begin//wait for start button press
debounce();
if (New_Butnum)
begin
switch(Current_Butnum)
begin
case Btn_Start:
lcd_gotoxy(0,1);lcd_putsf("Start");
done=1;
break;
default:
break;
end//end switch
Clr_Butnum;
end
delay_ms(30);
end
//S_Printf("Writing run\n\r");
STA013_Write(0x72,0x01);
delay_us(2);
//S_Printf("Writing play\n\r");
STA013_Write(0x13,0x01);
delay_us(2);
//S_Printf("Writing mute\n\r");
STA013_Write(0x14,0x00);
//DATA_REQ should go high
X_Start_Instant();
X_Poll();//this runs until transfer complete
STA013_Audio_Stop();
S_Printf("File transfer complete\n\r");
lcd_clear();
lcd_putsf("Btn 0 to start");
//X_Show_Buffer();
end
end
void initialize(void)
begin
lcd_init(16);//16 char LCD
lcd_clear();
lcd_gotoxy(0,0);
lcd_putsf("Serial MP3 v1.0");
Init_Serial();//intialize Serial I/O
#asm
sei
#endasm
UI_Init();//User interface
//Test UART communication
S_Printf("Serial MP3 player v1.0 by Zhi-Hern Loh\n\r");
//Init_Xmodem();//intialize Xmodem state vars
end |