Our project is an expandable bar inventory system
that implements wireless communication.
The bar inventory system was an interesting project,
because it involved both hardware and software together, since we are
comprised of one analog designer and one computer programmer, both of whom
are alcoholics. On the hardware side, there are sensors and wireless
communication, and on the software side, there is data encoding and clever
data manipulation.
Also, it was an excuse for us to drink for class, and
not because of class.
The project idea sprang from the concept of smart
refrigerators that keep an inventory of their contents. However, we feel that
alcohol is more important than food, so we wanted to make sure we didn’t
run low at any time. Therefore, slowly the project morphed into an
inventory system for commercial use in a bar. The project was intended to
be adjustable and convenient for any bar.
Some background math was necessary, because we wanted
the system to be practical. It is not practical for users of the system to know the exact mass of
a liquor bottle, so a scheme was developed that would require just
an empty bottle and a full bottle to calculate both a minimum and maximum
“weight” for the bottles. The weights were actually just the values
obtained from the ADC, since actual mass units were not necessary. These
extrema allowed us to calculate percentages:

Note: The force sensor decreases
resistance with increased force, and thus the MinWeight is larger than
the MaxWeight.
One hardware tradeoff dealt with using the UART on
the ATMEL Mega16L microcontroller on the receiver side. The UART had to
be reserved for serial communication with a HyperTerminal, so it could not
be used to aid in the wireless communication. This also affected the
software, because the necessary code for RF transmission became rather
tedious and extremely specialized.
The largest tradeoff we had involved communication
with a television display. Initially we wanted to be able to display the
inventory status on a TV screen, but there were so many synchronization
issues involving the RF transmission (which itself had its own timing
issues because it was transmitting two different pieces of information)
and the serial communication. The main problem was that since we couldn't use UART for wireless transmission, we needed to manually transmit bits on the order of 1 per millisecond, which is much faster than the clock used for the
TV. So, to implement both the wireless and the TV, we would need two timer interrupts: one for the
TV and one for wireless. This turns out to be impossible at the level of accuracy required for both of these devices, since multiple interrupts cannot be processed at the same time. It also would affect our
budget since we would be required to use a Mega32 as opposed to a cheaper
Mega16. Finally, we decided that the output could just easily be
outputted onto HyperTerminal without really taking away from the visual
experience, because it was primarily a text display.
The final project block diagram can be found in
Appendix B.
Because we are using RF transmission, we are required to comply with the
FCC regulations described in Title 47, Chapter 1, Part 15. Quoting from
Section 15.5(b), “Operation of [a]…radiator is subject to the conditions
that no harmful interference is caused and that interference must be
accepted that may be caused by the operation of an authorized…radiator.”
Also, since our device is a home-built device (i.e. it’s not marketed,
constructed from a kit, or built in quantities greater than 5, and is for
personal use), we are not required to get a license for operation and meet
regulations exactly. We are, however, required to “employ good
engineering practices” (47CFR15.23).
We are not aware of any inventory system for a bar, and we don’t know of
any copyright and trademarks that we are infringing upon. We do however
say that any pictures involving alcohol bottles are trademarks of their
respective liquor companies, however, the pictures are copyrighted to us.
In building the inventory system, we used a force
sensor to obtain the weight of a bottle. The bottle rests on a stand
which pushes down on the force sensor and varies the resistance between
its two leads. This variable resistance was then translated into a
variable voltage using a simple voltage divider circuit. Resistors with a
ratio of 5:1 were used in the divider to allow the voltage swing to be
reasonable. This voltage is fed into the analog-to-digital converter
(ADC) of an ATMEL Mega163 microcontroller, therefore we need the input
swing to be large enough to interact with the internal voltage reference
of the ADC.
There were two bottle stations so we had two inputs into the ADC, but
only one output, which was controlled by the ADMUX register on the MCU.
There was a slight hurdle with this: if the ADMUX register was
changed while a conversion was occurring, there we be incorrect
operation. To correct this, we had to look at the ADIF bit in the ADCSR
register. When ADIF is high, the conversion is complete, and so we can
then change the value of ADMUX.
The ADC output was then encoded and sent to a
transmitter operating at 433 MHz.
The transmitter used a choke inductor and blocking capacitor to the power
supply from the PC board power supply to allow for a larger range on
transmission. The choke inductor helps to eliminate power supply
noise, and allows for a more DC stable power supply into the transmitter.
The choke inductor was just wire wrapped around a regular pen to create an
inductor on the order of μH. I also placed a blocking capacitor
between the antenna output and the antenna, which was a quarter wave
length (≈17 cm) piece of wire. Since the receiver takes some time to
turn on, we first transmit 4 ‘a’s followed by a start bit (2 1's) to indicate the
start of data. A Manchester encoding scheme was used for the data to
allow for the receiver to keep the correct auto-gain settings. The
receiver will increase and decrease its gain depending on whether or not
it received a lot of 0s or 1s. Therefore, over a given number of bits, we
want the numbers of 0s and 1s to be the same. Manchester encoding is a
simple way to do this since each bit is encoded into 2 bits—one 1 and one
0. Though Manchester encoding is a bit conservative, we transmit
so little data that we could be lax on this. Furthermore, Manchester encoding provides additional data integrity checks, which allow the receiver to be quite confident that the data obtained is what the transmitter sent.
Transmission was complicated because it had to
transmit from two different bottle stations. So, each transmission
included start sequences, bottle station identifiers, and weight values.
This complexity made the expandability of the system convenient because bottle stations transmit independently (rather than, say, transmitting information about both bottles in one chunk). It
does not matter when a transmission is sent, just that it is sent at all. To add more bottle stations, no additional work needs to be done with respect to the transmission units.
We also have a delay between transmissions, so we won’t have to worry
about the transmissions running into each other on the receiver side.
To further complicate things, we were unable to use
the UART for RF transmission, since it had to be reserved for serial
communication to a computer on the receiver end. Since we would only be
allowed to use the UART on one end, we felt it would be easier to not use
it on both the transmitter and receiver sides.
The receiver, contained on its own custom PC board,
then passed the data to an ATMEL Mega16L microcontroller. The MCU does
various checks on the data before it can accept it as valid. First, it
must check for a minimum of 4 ‘a’s (alternating high and low), and once it recognizes the start bit,
the MCU stores the data into a
transmit_buffer.
While the MCU is receiving data, it is checking for Manchester encoding
(i.e. checking to see if the odd-index bits received are the complement of the event bits). This allows us to ignore transmissions that may have lost a bit
in communication or garbage received due to random noise or other transmitting devices. Once the data passes checking, the MCU decodes it, and
updates the inventory. However, we don’t want the inventory to update if
a bottle is removed from a station to be poured into a glass. To prevent
this update, if a bottle station receives a weight that is less than the
empty bottle weight, the current weight will not be updated.
The MCU also contains the user interface which
interacts with the HyperTerminal. There was a menu with functional
commands including: 1) programming a bottle station, 2) adding a bottle
selection, 3) removing a bottle selection, 4) checking the inventory, 5)
displaying the bottle list, and 6) resetting all the settings. A detailed
list of the functions is as follows:
1) Program
a bottle station: Programs one of the weight stations with a bottle
chosen from the bottle list with preprogrammed bottles. A bottle can also
be removed from the station.
2) Add
a bottle type: Adds a type of bottle to the existing bottle list.
This can only be done on the designated program station and requires both
an empty and a full bottle. This way both a minimum and maximum weight
can be obtained to calculate weight percentages with various liquid
levels. A maximum volume is also prompted to enable shot calculation
(calculate number of shots remaining).
3) Remove
a bottle type: Removes a bottle from the existing bottle list. This
allows one bottle to be removed at a time, as opposed to resetting all
settings. This also warns the user if they delete a bottle which is
currently programmed onto a station, but it still allows removal.
4) Check
the inventory status: Displays the remaining percentage in each
bottle as well as the number of shots remaining in the bottle.
5) Display
the bottle list: Displays the existing bottle list.
6) Reset
all settings: This command is necessary since we save the bottle list
along with programmed bottle stations in EEPROM.
There was one minor detail involving EEPROM because
you can’t store pointers in EEPROM. This meant we were unable to use
sprintf, which is
typically used for copying character arrays. We solved this by creating
our own character array copier that copies characters one by one into a
separate character array.
As mentioned above, we initially tried to use the TV
as a display, but were unsuccessful. We also tried to improve the
accuracy of the inventory with an exponential decay weighting scheme to make weight readings
more accurate and stabilize faster. This didn’t really work, because it
just made the stabilization take longer, since older values were incorrect
and should simply be replaced with new readings.
We are pleased to say that we managed to get the
project to work and function as intended. There are some time delays with
bottle calibration, because adding a bottle requires a long time period
for accurate readings. However, this is not too inconvenient in a real
life setting, because the bottle needs only to be calibrated once.
The accuracy is not always consistent because of
settling time on the force sensor. However, the longer time waited for
bottle calibration, the better the accuracy. Also, we noticed that the
sensor sensitivity varied for different weights, which affected accuracy.
This was unexpected since the data sheet showed a linear resistance vs.
mass relationship.
To our joy, RF Transmission was very accurate. Because of our thorough
data checks, invalid data was hardly ever accepted, and if it was, it was
completely replaced with the next transmission. We transmitted every half
a second, so updating was accurate enough for practical application.
(1) According to the surgeon general, women should
not drink alcoholic beverages during pregnancy because of the risk of
birth defects. (2) Consumption of alcoholic beverages impairs your
ability to drive a car or operate heavy machinery, and may cause health
problems.
We also do not condone underage drinking.
Since liquid is involved in this project, we had to ensure the
electronics would not be harmed and cause electrocution. Since in a real
life situation, a bartender may spill while pouring, the exterior of a
bottle may get wet so the weight stations have a plastic top to save the
force sensor from getting wet. Ideally we would also want to encase the
transmitter PC board, however I was not able to dig around my room enough
to find something that would work and not interfere with RF communication.
Though we did not try to transmit and receive while others were
transmitting, it is possible that our project may not receive accurate
readings as often as it normally would. The MCU will still ignore data
that is not valid, which is likely to occur with interference, so the
inventory should not alter dramatically. However, this was never actually
tested.
Usability was an important feature of the project because we wanted it
to be useful in a practical application. The user interface is easy to
use and incorporates many functions. Also the method of programming a
bottle was designed to allow for little knowledge necessary of the actual
bottle.
The design met our expectations, however the accuracy was a little random
because of the pressure sensors. The RF transmission worked better than
expected, mostly due to data validation. The user terminal was very
reliable and allowed for mistakes to be corrected without resetting the
program. Also, terminal use is non-blocking with the inventory updating.
Next time, we might use more than one pressure sensor for each bottle
station to improve accuracy. Or, we may try to find a different way of
sensing pressure that used a different type of sensor.
1. ...to be honest and realistic in stating claims
or estimates based on available data;
We honestly state the results
of our project by reporting both the good points and the bad points.
2. ...to reject bribery in all its forms;
We will openly share our design
with anyone who requests information and wants to replicate the project.
We will accept donations, but not bribes.
3. ...to seek, accept, and offer honest criticism
of technical work, to acknowledge and correct errors, and to credit
properly the contributions of others;
We will definitely accept any
criticism of the project, especially if it is paired with suggestions for
improvement on the design. Credit would be given to the proper sources of
information.
4. ...to avoid injuring
others, their property, reputation, or employment by false or malicious
action;
We don’t intend harm on anyone
or their employment. Therefore we warn everyone that alcohol may impair
your judgment and should not be consumed excessively.
5. ...to assist colleagues and co-workers in their
professional development and to support them in following this code of
ethics.
We gladly assist colleagues in
the common goal of learning and applying knowledge gained in a practical
design.

Final Project Block Diagram

Voltage Divider Circuit

Transmitter Pinout and Circuitry
We came just under budget, however we could have saved on power had we
attached batteries instead of using power supplies. We also could
have saved money by sampling earlier than we did
Part |
Quantity |
Price |
Force
Sensor |
2 |
$9.50
($4.75 each) |
ATMEL
Mega16L |
1 |
$5 |
ATMEL
Mega163 |
1 |
$2 |
Custom PC Board |
1 |
$5 |
Custom PC Board w/ RS232 |
1 |
$10 |
Power
Supply |
2 |
$10 |
Transmitter/Receiver Pair |
1 |
$8 |
White
Boards |
2 |
Free
(previously owned) |
Pepsi
Bottle Caps |
6 |
Free
(previously owned) |
Cheap
Cutting Board |
1 |
Free
(previously owned) |
Bottles |
3 |
Free
(previously owned) |
Personal Computer w/ HyperTerminal |
1 |
Free (previously owned) |
|
Total: |
$49.50 |
We both worked on everything simultaneously. However, Adam has more
knowledge into the exact inner workings of the code, whereas Tania has more
knowledge of the operation of the hardware. Though we both understand all
aspects of the design.
ECE 476 Final Project: Demolition RC car,
http://instruct1.cit.cornell.edu/courses/ee476/FinalProjects/s2004/cml45/webpage/index.html
by Christopher Lehmann, Steve Lowe, and Daren Zou

Picture of us simulating our vision once the
bottle is empty.
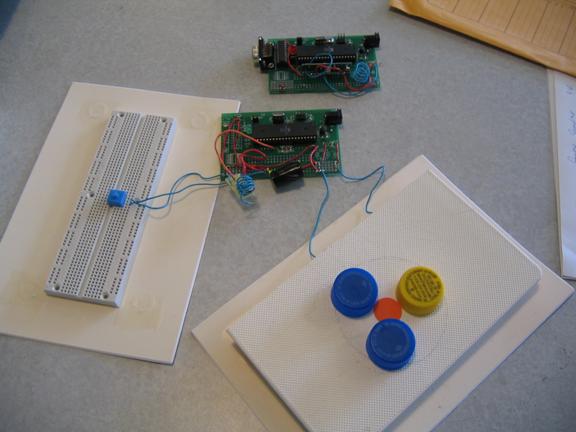

Complete Labeled System