Project Deidre: Usage of DX macros
I. Muscle Primitives
The foundation of this project on modeling human facial expressions is
the muscle primitives. To check out the details of how the muscle primitives
work, click here.
a. Linear Muscle
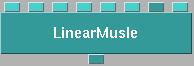
Click here to download the
Linear Muscle module...
The linear muscle primitive distributes displacement vectors
to all vertices on a skin patch. The vectors' directions are the
same for all vertices, however, magnitude varies inversely (in a smooth
sine fashion) according to each individual vertex's distance to the
point of greatest displacement as defined by user (Pd).
The inputs are as follows:
- Po - Origin point of greatest displacement on the skin patch.
(Or the original coordinates of the point on the skin patch that will move
the most.)
Format: DX vector [x,y,z]
- Pd - Ending point of greatest displacement on the skin patch.
(Or the ending coordinates of the point on the skin patch that will move
the most.)
Format: DX vector [x,y,z]
- SurfaceMesh - Face Mesh to be stretched.
- BBox1 - First corner point of the bounding box on the skin mesh.
Note that BBox1 and BBox2 should have the property that either the
expression
(BBox1.x > BBox2.x) and (BBox1.y > BBox2.y)
and (BBox1.z > BBox2.z)
is true, or the expression
(BBox1.x < BBox2.x) and (BBox1.y < BBox2.y)
and (BBox1.z < BBox2.z)
is true.
Format: DX vector [x,y,z]
- BBox2 - Second corner point of the bounding box on the skin mesh.
Note that BBox1 and BBox2 should have the property that either the
expression
(BBox1.x > BBox2.x) and (BBox1.y > BBox2.y)
and (BBox1.z > BBox2.z)
is true, or the expression
(BBox1.x < BBox2.x) and (BBox1.y < BBox2.y)
and (BBox1.z < BBox2.z)
is true.
Format: DX vector [x,y,z]
- Radius - affects both the curvature of the stretched skin patch and
the area of skin affected. Should usually be larger than the distance from
Pd to the furthest edge on the affected skin patch.
- frac - Sequencer fraction (i.e. should be a fraction between
0 and 1 depending on sequencer number). For example, for a ten frame animation
should be .1 at first sequencer frame and 1.0 at the tenth sequencer frame.
- OrigMus - Original Mesh - the original (unstretched) surface. Note
that this must be the facial mesh before any muscle vectors were applied to
the mesh. It's used in the magnitude calculation of the vectors.
The module outputs the DX object inputed in Mesh stretched accordingly.
b. Sphincter Muscle
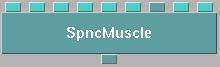
Click here to download the Sphincter
Muscle module...
The sphincter muscle primitive distributes displacement vectors
to all vertices on a skin patch. The vectors' directions are either
toward or away from a user defined point (Po). Magnitude varies proportionally
(in a smooth sine fashion) according to each individual vertex's distance to the
"center" of the sphincter muscle as defined by user (Pd). The inputs are as
follows:
- Po - "Center" of the sphincter muscle. The point which all other
points move toward or away from.
Format: DX vector [x,y,z]
- Pd - "Destination" of the sphinter mucle. Affects the direction of
other points (could be the same as Po for a flat 2D sucked in effect).
Format: DX vector [x,y,z]
- SurfaceMesh - Face Mesh to be stretched.
- BBox1 - First corner point of the bounding box on the facial mesh.
Note that BBox1 and BBox2 should have the property that either the
expression
(BBox1.x > BBox2.x) and (BBox1.y > BBox2.y)
and (BBox1.z > BBox2.z)
is true, or the expression
(BBox1.x < BBox2.x) and (BBox1.y < BBox2.y)
and (BBox1.z < BBox2.z)
is true.
Format: DX vector [x,y,z]
- BBox2 - Second corner point of the bounding box on the facial mesh.
Note that BBox1 and BBox2 should have the property that either the
expression
(BBox1.x > BBox2.x) and (BBox1.y > BBox2.y)
and (BBox1.z > BBox2.z)
is true, or the expression
(BBox1.x < BBox2.x) and (BBox1.y < BBox2.y)
and (BBox1.z < BBox2.z)
is true.
Format: DX vector [x,y,z]
- Radius - affects both the curvature of the stretched skin patch and
the area of skin affected. Should usually be larger than the distance from
Pd to the furthest edge on the affected skin patch.
- frac - Sequencer fraction (i.e. should be a fraction between
0 and 1 depending on sequencer number). For example, for a ten frame animation
should be .1 at first sequencer frame and 1.0 at the tenth sequencer frame.
- Adj - A [x,y,z] vector determining the "toward center" or "away from center" effect of the sphincter muscle. A negative number denotes "toward
center," a positive number denotes "away from center" and a zero denotes
"no effect" in either x, y, or z direction.
Format: DX vector [x,y,z]
The module outputs the DX object inputed in Mesh stretched accordingly.
c. Examples using Muscle Primitives
Linear Muscle Example
This is an example using linear muscle. The linear muscle has the following
input values:
Inputs
| Values
|
Pd
| [334 -548 -710]
|
Po
| [334 -530 -710]
|
SurfaceMesh
| Face (the Deidre face mesh)
|
BBox1
| [255 -599 -700]
|
BBox2
| [255 -535 -600]
|
Radius
| 110
|
frac
| Ten frames, values goes from .1, .2, ... 1.0
|
OrigMus
| Face (the Deidre face mesh)
|
The networks looks as follows:
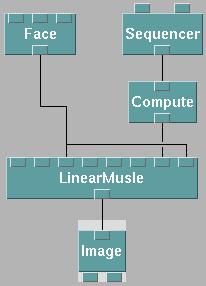
Here is the result (taken as sequencer fraction = 1.0)
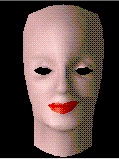
Notice the slight grin cause by the stretching of the lip corner via a
linear muscle.
Sphincter Muscle Example
This is an example using sphincter muscle. The sphincter muscle has the
following input values:
Inputs
| Values
|
Pd
| [256 -550 -646]
|
Po
| [256 -550 -620]
|
SurfaceMesh
| Face (the Deidre face mesh)
|
BBox1
| [106 -599 -700]
|
BBox2
| [412 -535 -600]
|
Radius
| 200
|
frac
| Ten frames, values goes from .1, .2, ... 1.0
|
OrigMus
| Face (the Deidre face mesh)
|
Adj
| [.85 -.25 .8]
|
The networks looks as follows:
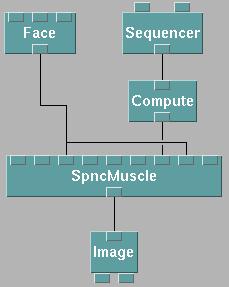
Here is the result (taken as sequencer fraction = 1.0)
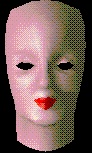
Notice the pouting (puckering up) of the lips as a result of the sphincter
muscle.
II. Emotion Macros
Building on top of the muscle primitives, we created six macros that
stretches the Deidre facial mesh into various emotion states. To see how
the six universal emotions are broken up into muscle primitives and
movies of our results, click AngerEyebrow.net
DisgustEyebrow.net
MadEyebrow.net
SadEyebrow.net
a. Happiness (smile)

Click here to download the smile module...
The smile module is simple, you basically plug in the facial mesh (any DX object)
and a sequencer output between 1 to 10 (representing frame number), and
the smile module output is the stretched smiling face.
b. Other Emotions
(Anger, Disgust, Fear, Surprise, and Sadness)

Click here to download the anger module...
Click here to download the disgust module...
Click here to download the fear module...
Click here to download the sadness module...
Click here to download the surprise module...
The anger, disgust, fear, sadness and surprise modules are structured the same
way. The first input is that of the facial mesh (any DX object), the second
and third inputs should be the eyebrows and the final input should be the
sequencer output between 1 to 10 (representing the frame number). The output
of the emotion modules is simple a facial mesh stretched to expression the said
emotion.
c. Examples using Emotion Macros
Emotion Macros Example
This is an example using emotion macros. The network is as follows:
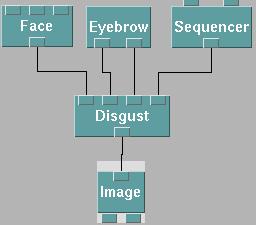
Here is the result (taken as sequencer output = 10)
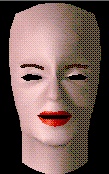
III. Facial Feature Constructs
As part of our project in modeling human facial expressions, we've
also managed to build a series of facial features that are not part
of the face mesh. This includes eyebrows, hair, tongue, eyes, etc.
Some of the more interesting constructs are provided as macros here.
Click here to download hair macro...
Click here to download eyebrow macro...
Back to Main Page.
Maintained by Dan Hung, last updated Apr. 27th 1996