The software design
The software in this project is two-fold; the controller, which receives inputs from the voice recognition chip and outputs to the TV, and the chess algorithm, which checks if the moves follow the rules of chess. These two algorithms must be run in the microcontroller simultaneously, so that the user does not go through any video distortions or other unwanted artifacts. In this section, we will talk about the big picture, then discuss the two parts separately in detail.
The Big Picture
Much of the original algorithm is based on that given by Bruce Land in a previous lab session. Since the video output required for the chess algorithm is very similar to that of the digital oscilloscope, we have managed to manipulate the algorithms to make a chessboard with pieces. Using these pictures, the user can now see where the pieces are on the board.
On another section, the microcontroller controls the voice inputs and tries to understand what the user is saying. Through the HM2007, it finds what the voice recognition chip interprets the chip to be, and understands the user’s inputs.
Finally, the microcontroller itself has an algorithm that checks whether the command is valid or not. This exists for two reasons -- to make sure that each user has a fair chance at playing chess, and also to weed out possible misunderstanding of moves of the pieces. Also, it makes sure that it is possible to take back moves, just incase the move was still valid, but not what the user wanted it to be.
As a whole, the following diagram shows the general order of the state machine of the microcontroller.
Figure 7: Basic State Machine of Microcontroller
Using such a state machine, we can see that the microcontroller was created through three major states. We will examine these states in more detail.
Initialization
The initialization of the circuit takes two processes. First, the microcontroller initializes the chessboard array into a starting position onto the board, very much like Figure 8 below. After this, the microcontroller drives a state machine (explained further below) through the function void train(), where the original voice inputs are entered. This is predefined in the control code, where the user would be required to record their voice for future recognition. In addition, the TV shows the input number to verify if the microcontroller received the signal. Much of this code is specified in the main() function before the chess algorithm is implemented.
Placing pieces
Within the main function (inside the code), we have recreated the board through a 8x8 array, which has variables that specifies whether or not a piece is there, and if so, what piece from what side.
Figure 8: Picture of the Video Chess Board
However, the major problem is the board itself. Since we have two different colors of pieces (black, white) and two colors in the checkerboard (black, white), we have four different kinds of displays (black piece on white square, black on black, white on white, and white on black) for each of the six pieces available (pawn, rook, knight, bishop, king, queen). These pieces are defined a specific value on the chessboard array based on the piece’s alphabetical order, thus a black spot would be 0, bishop 1, king 2, knight 3, pawn 4, queen 5, and rook 6.
In order to insure that all the pieces are placed with the proper notation, we used some simple code to indicate the color of the array. Using this data, we created a function within the main to distinguish a white (or black) pieces’ background color, and appropriately assign the right symbol in the right location.
As shown in the previous lab, the video board itself uses the same type of functions to display onto video. Functions such as void video_pt(char x, char y, char c), void video_putchar(char x, char y, char c), void video_puts(char x, char y, char c), void video_putsmalls(char x, char y, char *str), and void video_line(char x1, char y1, char x2, char y2, char c) were used to properly display the chessboard.
Receiving Voice Commands
Once the board is displayed, we receive voice commands from the user translated (and encoded) by the HM2007. These voice commands, which are inputted previously in a specific order, outputs their respective number according to the sound that has been inputted. However, the chip requires a series of control codes given by the microcontroller.
Figure 9: Circuit Diagram for Microcontroller Usage
The HM2007 does several different functions: recognition, which identifies the specific speech segment; resulting, which outputs the results; training, which lets the user input the result; uploading, which uploads an existing voice file; and downloading, which downloads a file within the HM2007. For the usage of this project, we require to run the recognition, resulting, and training input codes. 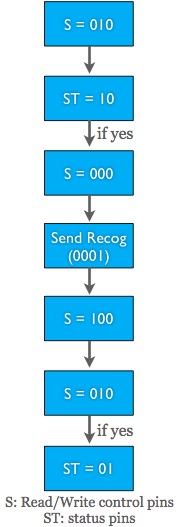
The right (Figure 10: Sample State Diagram, Recognition), shows an example of how the microcontroller controls the voice recognition chip to create an appropriate output. Therefore, within our code, we have created three different functions, void recog(), void result(), and void train() to manage these processes. These functions were created based on state diagrams on the datasheet. Also, to ease and streamline the process of receiving pieces and enabling the initialization process, we also created another function, void initialize() . These function is essentially an application of the previous three command codes: it creates the process of receiving the user’s input into the program for the appropriate pieces and locations.
Validity Check
Using the recognized inputs, the code then goes into a validity check. This code goes through the examination of two aspects of the inputted command, the original location and the location that it will be going to. Using this data, we can find information on whether such a piece really exists, and if so, if that piece can actually go to that location.
The validity check is important because we want to make sure that it does not take very long to execute; however, since chess pieces move almost randomly, we have to consider many different situations. For example, a pawn generally only moves forward one step, but depending on its situation and location, it can go forward two steps, go forward one step and horizontally one step, or even change into a new piece. As a result, we wrote this code in the form of a switch function that can check if the movement is valid or not. The checking of the original location is quite easy; however it is essential to know in order to check whether the move is valid or not.
In addition to this validity check, the microcontroller must also assess the situation on the board. If the king is in check, the user is notified and must move accordingly. If the king is captured, the game must know that it is at the end. Such execution of code was done through an extensive combination of status statements, checking each specific situation of the piece in question.
This validity check (and the change of the chess array) occurs within void getmove(). The switch function first checks if the piece is actually in place and also if it is accessed by the correct side. Once this is checked, it goes through the kind of piece it is and verifies if the move is valid. If the move is valid, it also saves the that move in case of a move-back command. Using these techniques, we were able to create moves.